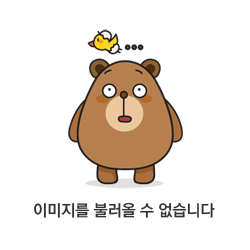
In this class, I learn the bitwise shift operator including '<<' and '>>'.
1. Right-shift operator (>>)
- The right-shift operator (<<) moves the bits of an integer or enumeration type expression to the right.
#include <stdio.h>
#include <stdlib.h>
/* run this program using the console pauser or add your own getch, system("pause") or input loop */
int main(int argc, char *argv[]) {
int A = 0b1000;
A = A >> 2;
printf('%x', A);
return 0;
}
The results showed 'A' as 0b0010 that which resulted in change of 2^(-2).
2. Left-shift operator (<<)
- The left-shift operator (<<) moves the bits to the left.
#include <stdio.h>
#include <stdlib.h>
/* run this program using the console pauser or add your own getch, system("pause") or input loop */
int main(int argc, char *argv[]) {
int A = 0b0001;
A = A << 2;
printf('%x', A);
return 0;
}
The results showed 'A' as 0b0100 that which changed by the square of 2.
'Study Group > 빡공단 30기' 카테고리의 다른 글
[Day 12] Conditional Statement 2: switch - case (0) | 2023.01.12 |
---|---|
[Day 11] Conditional Statement 1: if and else (0) | 2023.01.11 |
[Day 9] Operator 2: Logical Operators and Bit operators in C Language (0) | 2023.01.10 |
[Day 8] Operator 1: Assignment, Relational, Arithmetic, Incremental Operators in C Language (0) | 2023.01.08 |
[Day 7] Quadratic expression for C Programming (0) | 2023.01.07 |